Mega Millions Lottery Ticket Checker
Introduction
Currently, I am developing a Flutter based mobile application that will quickly scan lottery tickets and determine winning tickets, and how much they won by. To get started, I wanted to create a proof of concept of the backend process to check a set of numbers against the last winning numbers, and determine the prize amount. To do this, I wrote a library in Python, which I will later convert to Dart, or perhaps an API endpoint.
Before we get to far, it is important to recognize that I am not affiliated with the Mega Millions Lottery, Mega Millions is copyright and all rights reserved by Mega Millions.
The Process
The process can be described with the following steps:
1.) Get the current lottery information. This includes the current winning numbers, the megaplier amount, the megaball number, and the jackpot amount.
2.) Determine the number of matches
3.) Calculate the prize table, and lookup the prize
How To Get the Current Lottery Information
In order to get the lottery information, I began by searching for APIs that provide the information I needed. One such I found is hosted on RAPID API: https://rapidapi.com/avoratechnology/api/mega-millions.
I didn’t really like the pricing model, so I decided to check the mega millions website for the datasource that is used to display the dynamic content on the page. This was straightforward and easy to do. By using Chrome Developer Tools (F12), you can see how a page loads, and what resources it accesses. Here’s what the Mega Millions site uses:
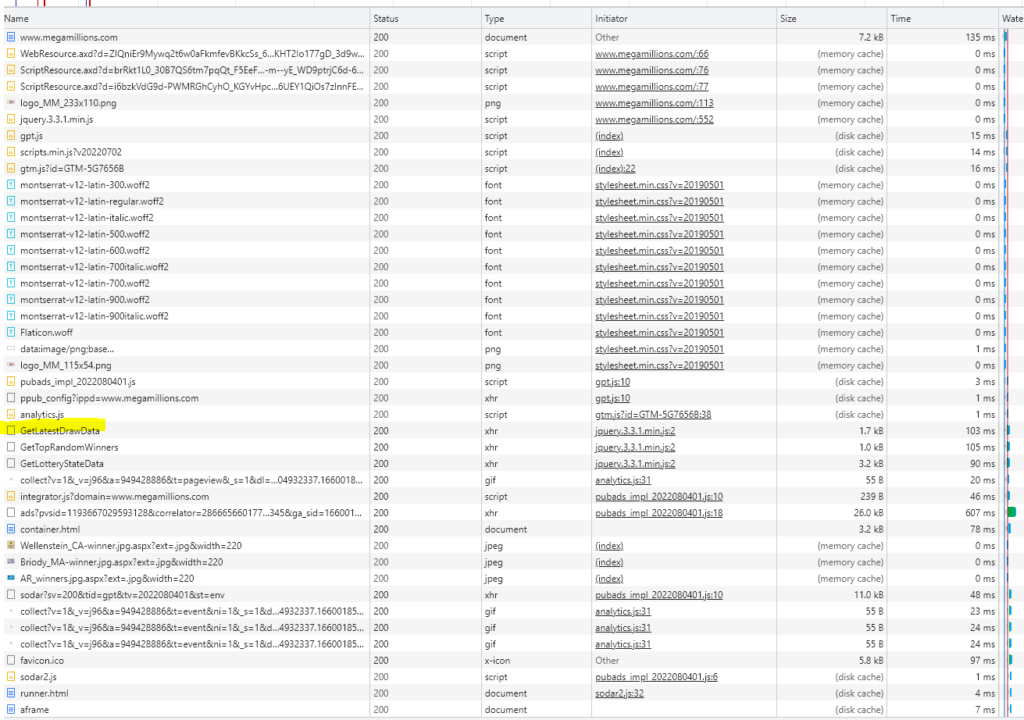
GetLatestDrawData
is interesting! Let’s take a look. Double click on the file to see the URI’s content:
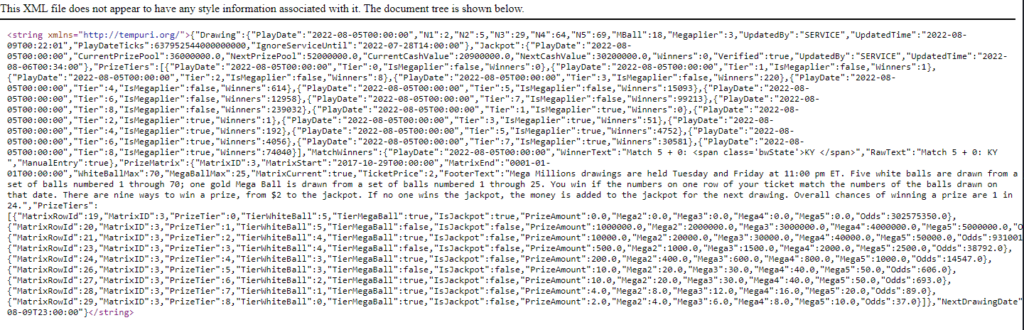
Yeah, that looks usable.
We can see a JSON that is wrapped in XML. We can very simply write a script to extract the fields that we need. To do this:
1.) Make a request for the data
2.) Strip the XML Padding using regex.
3.) Convert the remaining information to a JSON dict.
4.) Return a JSON of the fields we care about
Here’s the code:
from urllib.error import HTTPError
from config import MEGAMILLIONS_URL
import requests
import json
def strip_xml(string_to_strip):
return re.sub('<[^<]+>', '', string_to_strip)
def get_latest_numbers():
try:
http_results = requests.get(MEGAMILLIONS_URL).text
except:
raise HTTPError
json_results = json.loads(strip_xml(http_results))
drawing_details = json_results['Drawing']
return {"PlayDate": drawing_details['PlayDate'],
"Numbers": [drawing_details['N1'],
drawing_details['N2'],
drawing_details['N3'],
drawing_details['N4'],
drawing_details['N5']],
'MegaBall': drawing_details['MBall'],
'Megaplier': drawing_details['Megaplier']}
And here’s code to extract the lottery pool, using similar methods:
def get_latest_prize():
try:
http_results = requests.get(MEGAMILLIONS_URL).text
except:
raise HTTPError
json_results = json.loads(strip_xml(http_results))
drawing_details = json_results['Jackpot']['CurrentPrizePool']
return drawing_details
Determine the Winning Amount / Prize Pool
We will use a hash table to lookup the prizes. We can do this by using unique keys. m
describes a matching MegaBall, n
describes a non match. The number following this, designates the number of matching balls. So, a key for a matching MegaBall and 3 balls would have the key m3
.
With the megaplier, we need to adjust the prize table by a factor of the current megaplier. We can simply multiply each value in the hash table by the megaplier, then proceed with regular lookup of prize.
Here’s the Code:
def determine_prize(ticket_numbers, ticket_megaball, ticket_megaplier=False):
latest_drawing = get_latest_numbers()
latest_prize = get_latest_prize()
winning_numbers = latest_drawing['Numbers']
megaball = latest_drawing['MegaBall']
megaplier = latest_drawing['Megaplier']
matches = 0
for number in ticket_numbers:
if number in winning_numbers:
matches+=1
prize_table = {'n0': 0, 'm0': 2, 'n1':0, 'm1': 4, 'm2': 10, 'n2': 0, 'n3':10, 'm3': 200, 'n4': 500, 'm4': 10000, 'n5': 1000000, 'm5': latest_prize}
if ticket_megaplier == True:
for key, value in prize_table:
prize_table[key] = value * megaplier
if megaball == ticket_megaball:
lookup_key = 'm'
else:
lookup_key = 'n'
lookup_key += str(matches)
return prize_table[lookup_key]
Complete Code
from urllib.error import HTTPError
from config import MEGAMILLIONS_URL
from util import strip_xml
import requests
import json
def get_latest_numbers():
try:
http_results = requests.get(MEGAMILLIONS_URL).text
except:
raise HTTPError
json_results = json.loads(strip_xml(http_results))
drawing_details = json_results['Drawing']
return {"PlayDate": drawing_details['PlayDate'],
"Numbers": [drawing_details['N1'],
drawing_details['N2'],
drawing_details['N3'],
drawing_details['N4'],
drawing_details['N5']],
'MegaBall': drawing_details['MBall'],
'Megaplier': drawing_details['Megaplier']}
def get_latest_prize():
try:
http_results = requests.get(MEGAMILLIONS_URL).text
except:
raise HTTPError
json_results = json.loads(strip_xml(http_results))
drawing_details = json_results['Jackpot']['CurrentPrizePool']
return drawing_details
def determine_prize(ticket_numbers, ticket_megaball, ticket_megaplier=False):
latest_drawing = get_latest_numbers()
latest_prize = get_latest_prize()
winning_numbers = latest_drawing['Numbers']
megaball = latest_drawing['MegaBall']
megaplier = latest_drawing['Megaplier']
matches = 0
for number in ticket_numbers:
if number in winning_numbers:
matches+=1
prize_table = {'n0': 0, 'm0': 2, 'n1':0, 'm1': 4, 'm2': 10, 'n2': 0, 'n3':10, 'm3': 200, 'n4': 500, 'm4': 10000, 'n5': 1000000, 'm5': latest_prize}
if ticket_megaplier == True:
for key, value in prize_table:
prize_table[key] = value * megaplier
if megaball == ticket_megaball:
lookup_key = 'm'
else:
lookup_key = 'n'
lookup_key += str(matches)
return prize_table[lookup_key]
Link to Repository: https://github.com/psidney/lotto-checker